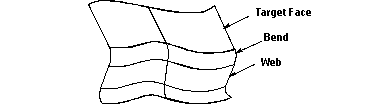
Figure Two Step General Flange
This example requires a blank, open part. The code creates a block
and a general flange.
#include <stdio.h>
#include <string.h>
#include <uf.h>
#include <uf_part.h>
#include <uf_curve.h>
#include <uf_csys.h>
#include <uf_defs.h>
#include <uf_modl.h>
#define UF_CALL(X) (report( __FILE__, __LINE__, #X, (X)))
static int report( char *file, int line, char *call, int irc)
{
if (irc)
{
char messg[133];
printf("%s, line %d: %s\n", file, line, call);
(UF_get_fail_message(irc, messg)) ?
printf(" returned a %d\n", irc) :
printf(" returned error %d: %s\n", irc, messg);
}
return(irc);
}
static void do_ugopen_api(void)
{
char *part_name = "general_flange";
int reverse_normal, poly_cubic, reverse_thicken;
int nsteps;
char *edge_lens[] = {"100.0","100.0","10.0"};
char thickness_str[132];
char tolerance_str[132];
double origin[3]={0.0,0.0,0.0};
UF_MODL_parm_t plus;
UF_MODL_parm_t radius[2], angle[2], length[2];
tag_t block_tag, target_face, flange_obj_id;
tag_t part, edge1_tag;
UF_STRING_t bend_edge, spine;
uf_list_p_t edge_list;
uf_list_p_t face_list;
UF_FEATURE_SIGN sign = UF_NULLSIGN;
UF_CALL(UF_PART_new(part_name, UF_PART_ENGLISH, &part));
UF_CALL(UF_MODL_create_block1 ( sign, origin, edge_lens,
&block_tag));
UF_MODL_ask_feat_faces ( block_tag, &face_list);
UF_MODL_ask_list_item ( face_list, 4, &target_face);
UF_MODL_ask_face_edges ( target_face, &edge_list);
UF_MODL_ask_list_item ( edge_list, 2, &edge1_tag);
UF_MODL_init_string_list ( &bend_edge);
UF_MODL_init_string_list ( &spine);
UF_MODL_create_string_list (1, 1, &bend_edge);
UF_MODL_create_string_list (1, 1, &spine);
bend_edge.num = 1; /* number of items in string array
*/
bend_edge.string[0] = 1; /* number of items in id array */
bend_edge.dir[0] = (int) UF_MODL_CURVE_START_FROM_BEGIN;
bend_edge.id[0] = edge1_tag;
spine.num = 1;
spine.string[0] = 1;
spine.dir[0] = (int) UF_MODL_CURVE_START_FROM_END;
spine.id[0] = edge1_tag;
plus.method_type = UF_MODL_PARM_CONSTANT;
strcpy ( plus.defined_by.constant.value, "0.0");
nsteps = 2;
radius[0].method_type = UF_MODL_PARM_CONSTANT;
angle[0].method_type = UF_MODL_PARM_CONSTANT;
length[0].method_type = UF_MODL_PARM_CONSTANT;
radius[1].method_type = UF_MODL_PARM_CONSTANT;
angle[1].method_type = UF_MODL_PARM_CONSTANT;
length[1].method_type = UF_MODL_PARM_CONSTANT;
strcpy ( radius[0].defined_by.constant.value, "25.0");
strcpy ( angle[0].defined_by.constant.value, "90.0");
strcpy ( length[0].defined_by.constant.value, "10.0");
strcpy ( radius[1].defined_by.constant.value, "30");
strcpy ( angle[1].defined_by.constant.value, "40.0");
strcpy ( length[1].defined_by.constant.value, "10.0");
reverse_normal = 0; /* =0 no change =1 flip it */
poly_cubic = 0; /* =0 rational =1
polynomial(approximate) */
reverse_thicken = 1; /* =0 along bend direction =1 opposite
bend dir */
strcpy ( thickness_str, "10.0");
strcpy ( tolerance_str, "0.01");
UF_CALL(UF_MODL_create_general_flange (target_face,
&bend_edge,
&spine, NULL, nsteps, poly_cubic, reverse_normal,
reverse_thicken, thickness_str, tolerance_str, &plus,
radius, angle, length, &flange_obj_id));
}
void ufusr(char *param, int *retcode, int paramLen)
{
if (!UF_CALL(UF_initialize()))
{
do_ugopen_api();
UF_CALL(UF_terminate());
}
}
int ufusr_ask_unload(void)
{
return (UF_UNLOAD_IMMEDIATELY);
}